■PHPでGoogle Cloud Vision APIにリクエスト
ここからは、サンプルプログラムにいきなり。
ズルく行きます。
参考は下記。
「Google Cloud Vision API(画像解析)を30分で試す」
http://qiita.com/t-fujiwara/items/7e1f7c52a73887519ac1
参考は下記。
「Google Cloud Vision API(画像解析)を30分で試す」
http://qiita.com/t-fujiwara/items/7e1f7c52a73887519ac1
このサンプルをそのまま借りてまず動くか?やってみます。
=======================
<?php
// APIキー
$api_key = “AIzaSyCKmmfDVoUhjGLyKaTBwTPncIbXd87VGWc” ;
<?php
// APIキー
$api_key = “AIzaSyCKmmfDVoUhjGLyKaTBwTPncIbXd87VGWc” ;
// 画像へのパス
$argv[1]=
$image_path = “$argv[1]” ;
$argv[1]=
$image_path = “$argv[1]” ;
// リクエスト用のJSONを作成
$json = json_encode( array(
“requests” => array(
array(
“image” => array(
“content” => base64_encode( file_get_contents( $image_path ) ) ,
) ,
“features” => array(
array(
“type” => “SAFE_SEARCH_DETECTION” ,
“maxResults” => 3 ,
) ,
) ,
) ,
) ,
) ) ;
$json = json_encode( array(
“requests” => array(
array(
“image” => array(
“content” => base64_encode( file_get_contents( $image_path ) ) ,
) ,
“features” => array(
array(
“type” => “SAFE_SEARCH_DETECTION” ,
“maxResults” => 3 ,
) ,
) ,
) ,
) ,
) ) ;
// リクエストを実行
$curl = curl_init() ;
curl_setopt( $curl, CURLOPT_URL, “https://vision.googleapis.com/v1/images:annotate?key=*****************************************************************” . $api_key ) ;
curl_setopt( $curl, CURLOPT_HEADER, true ) ;
curl_setopt( $curl, CURLOPT_CUSTOMREQUEST, “POST” ) ;
curl_setopt( $curl, CURLOPT_HTTPHEADER, array( “Content-Type: application/json” ) ) ;
curl_setopt( $curl, CURLOPT_SSL_VERIFYPEER, false ) ;
curl_setopt( $curl, CURLOPT_RETURNTRANSFER, true ) ;
if( isset($referer) && !empty($referer) ) curl_setopt( $curl, CURLOPT_REFERER, $referer ) ;
curl_setopt( $curl, CURLOPT_TIMEOUT, 15 ) ;
curl_setopt( $curl, CURLOPT_POSTFIELDS, $json ) ;
$res1 = curl_exec( $curl ) ;
$res2 = curl_getinfo( $curl ) ;
curl_close( $curl ) ;
$curl = curl_init() ;
curl_setopt( $curl, CURLOPT_URL, “https://vision.googleapis.com/v1/images:annotate?key=*****************************************************************” . $api_key ) ;
curl_setopt( $curl, CURLOPT_HEADER, true ) ;
curl_setopt( $curl, CURLOPT_CUSTOMREQUEST, “POST” ) ;
curl_setopt( $curl, CURLOPT_HTTPHEADER, array( “Content-Type: application/json” ) ) ;
curl_setopt( $curl, CURLOPT_SSL_VERIFYPEER, false ) ;
curl_setopt( $curl, CURLOPT_RETURNTRANSFER, true ) ;
if( isset($referer) && !empty($referer) ) curl_setopt( $curl, CURLOPT_REFERER, $referer ) ;
curl_setopt( $curl, CURLOPT_TIMEOUT, 15 ) ;
curl_setopt( $curl, CURLOPT_POSTFIELDS, $json ) ;
$res1 = curl_exec( $curl ) ;
$res2 = curl_getinfo( $curl ) ;
curl_close( $curl ) ;
// 取得したデータ
$json = substr( $res1, $res2[“header_size”] ) ; // 取得したJSON
$header = substr( $res1, 0, $res2[“header_size”] ) ; // レスポンスヘッダー
$json = substr( $res1, $res2[“header_size”] ) ; // 取得したJSON
$header = substr( $res1, 0, $res2[“header_size”] ) ; // レスポンスヘッダー
// 出力
echo “■出力結果” ;
echo $argv[1] ;
echo $json ;
echo “■出力結果” ;
echo $argv[1] ;
echo $json ;
=======================
はい、、ダメでした。。悲。。。。
なので、まず「ファイル指定」し、「ブラウザの制限」は無し、でやってみます。
phpファイル名は、「20170319_test1.php」
画像は、「富士山真上から」
画像は、「富士山真上から」
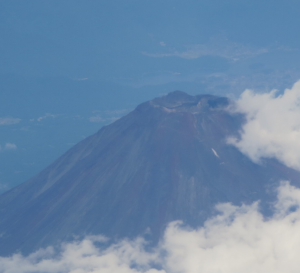
コードは、下記
=======================
<?php
$path = ‘/home/dasudasu/www/php_photo_20170318/files/IMG_0323.JPG’;
$result = curl_init();
// curl url
curl_setopt($result, CURLOPT_URL, “https://vision.googleapis.com/v1/images:annotate?key=********************************************************”);
// post
curl_setopt($result, CURLOPT_CUSTOMREQUEST, ‘POST’);
// –data-binary
curl_setopt($result, CURLOPT_BINARYTRANSFER, true);
// header
curl_setopt($result, CURLOPT_HTTPHEADER, array( “Content-Type: application/json” ));
// get response with text
curl_setopt($result, CURLOPT_RETURNTRANSFER, true);
// image file
$file_contents = base64_encode(file_get_contents($path));
$request = ‘{
“requests”: [
{
“image”: {
“content”: “‘. $file_contents .'”
},
“features”: [
{
“type”: “LABEL_DETECTION”
}
]
}
]
}’;
curl_setopt($result, CURLOPT_POSTFIELDS, $request);
// curl url
curl_setopt($result, CURLOPT_URL, “https://vision.googleapis.com/v1/images:annotate?key=********************************************************”);
// post
curl_setopt($result, CURLOPT_CUSTOMREQUEST, ‘POST’);
// –data-binary
curl_setopt($result, CURLOPT_BINARYTRANSFER, true);
// header
curl_setopt($result, CURLOPT_HTTPHEADER, array( “Content-Type: application/json” ));
// get response with text
curl_setopt($result, CURLOPT_RETURNTRANSFER, true);
// image file
$file_contents = base64_encode(file_get_contents($path));
$request = ‘{
“requests”: [
{
“image”: {
“content”: “‘. $file_contents .'”
},
“features”: [
{
“type”: “LABEL_DETECTION”
}
]
}
]
}’;
curl_setopt($result, CURLOPT_POSTFIELDS, $request);
// result
$response = curl_exec($result);
$response = curl_exec($result);
//結果表示
echo “</br>”;
echo $response ;
=======================
echo “</br>”;
echo $response ;
=======================
で、結果は、
{ “responses”: [ { “labelAnnotations”: [ { “mid”: “/m/01bqvp”, “description”: “sky”, “score”: 0.97879976 }, { “mid”: “/g/11jxkqbpp”, “description”: “mountainous landforms”, “score”: 0.9590976 }, { “mid”: “/m/0csby”, “description”: “cloud”, “score”: 0.9397293 }, { “mid”: “/m/0csh5”, “description”: “cumulus”, “score”: 0.92631036 }, { “mid”: “/m/01l56l”, “description”: “landform”, “score”: 0.90823483 } ] } ] }
おおおお???、「mountain」「sky」とか出てる?
(図1)
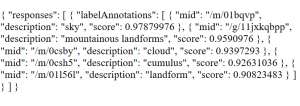
では、つなげてみるか。。。
=======================
<html>
<head>
<meta http-equiv=”Content-Type” content=”text/html; charset=utf-8″ />
<title>sample</title>
</head>
<body>
<p><?php
<html>
<head>
<meta http-equiv=”Content-Type” content=”text/html; charset=utf-8″ />
<title>sample</title>
</head>
<body>
<p><?php
if (is_uploaded_file($_FILES[“upfile”][“tmp_name”])) {
if (move_uploaded_file($_FILES[“upfile”][“tmp_name”], “files/” . $_FILES[“upfile”][“name”])) {
chmod(“files/” . $_FILES[“upfile”][“name”], 0644);
echo $_FILES[“upfile”][“name”] . ” をアップロードしました。</br>”;
echo $_FILES[“upfile”][“type”] . ” は、画像のMIMETYPEです。</br>”;
echo $_FILES[“upfile”][“tmp_name”] . ” は、サーバのフルパスです。</br>”;
echo $_FILES[“upfile”][“error”] . ” は、エラーコードです(=正常:0)。</br>”;
echo $_FILES[“upfile”][“size”] . ” は、画像のサイズです(bite)。</br>”;
if (move_uploaded_file($_FILES[“upfile”][“tmp_name”], “files/” . $_FILES[“upfile”][“name”])) {
chmod(“files/” . $_FILES[“upfile”][“name”], 0644);
echo $_FILES[“upfile”][“name”] . ” をアップロードしました。</br>”;
echo $_FILES[“upfile”][“type”] . ” は、画像のMIMETYPEです。</br>”;
echo $_FILES[“upfile”][“tmp_name”] . ” は、サーバのフルパスです。</br>”;
echo $_FILES[“upfile”][“error”] . ” は、エラーコードです(=正常:0)。</br>”;
echo $_FILES[“upfile”][“size”] . ” は、画像のサイズです(bite)。</br>”;
echo ‘<br />’;
//(1)このphpファイルのフルパス
echo “(1)このphpファイルのフルパス</br>”;
echo __FILE__ . ‘<br />’;
echo “</br>”;
echo “(1)このphpファイルのフルパス</br>”;
echo __FILE__ . ‘<br />’;
echo “</br>”;
//(2)このphpファイルのディレクトリパス
echo “(2)このphpファイルのディレクトリパス</br>”;
echo dirname(__FILE__) . ‘<br />’;
echo “</br>”;
echo “(2)このphpファイルのディレクトリパス</br>”;
echo dirname(__FILE__) . ‘<br />’;
echo “</br>”;
//(3)このphpファイルのスクリプト名
echo “(3)このphpファイルのスクリプト名</br>”;
echo basename(__FILE__) . ‘<br />’;
echo “</br>”;
echo “(3)このphpファイルのスクリプト名</br>”;
echo basename(__FILE__) . ‘<br />’;
echo “</br>”;
//(4)ローカルパス名、・・・でも表示不可能、残念
echo ‘(4)ローカルパス名、・・・でも表示不可能、残念<br />’;
echo $_POST[“filepath”]. ‘<br />’;
echo “</br>”;
echo ‘(4)ローカルパス名、・・・でも表示不可能、残念<br />’;
echo $_POST[“filepath”]. ‘<br />’;
echo “</br>”;
//(5)サーバ保管フォルダのフルパス名・・OK!
echo ‘(5)サーバ保管フォルダのフルパス名<br />’;
echo dirname(__FILE__) . “/files/” .$_FILES[“upfile”][“name”] . ” は、サーバ保管フォルダのフルパスです。</br>”;
echo “</br>”;
echo ‘(5)サーバ保管フォルダのフルパス名<br />’;
echo dirname(__FILE__) . “/files/” .$_FILES[“upfile”][“name”] . ” は、サーバ保管フォルダのフルパスです。</br>”;
echo “</br>”;
//(6)$argv[1]に合体させて表示、次phpにつなげる
echo ‘(6)$argv[1]に合体させて表示、次phpにつなげる<br />’;
$argv[1] = dirname(__FILE__) . “/files/” .$_FILES[“upfile”][“name”] ;
echo $argv[1];
echo ‘(6)$argv[1]に合体させて表示、次phpにつなげる<br />’;
$argv[1] = dirname(__FILE__) . “/files/” .$_FILES[“upfile”][“name”] ;
echo $argv[1];
}
} else {
echo “ファイルが選択されていません。”;
}
} else {
echo “ファイルが選択されていません。”;
}
$path = $argv[1];
$result = curl_init();
// curl url
curl_setopt($result, CURLOPT_URL, “https://vision.googleapis.com/v1/images:annotate?key=**********************************************************”);
// post
curl_setopt($result, CURLOPT_CUSTOMREQUEST, ‘POST’);
// –data-binary
curl_setopt($result, CURLOPT_BINARYTRANSFER, true);
// header
curl_setopt($result, CURLOPT_HTTPHEADER, array( “Content-Type: application/json” ));
// get response with text
curl_setopt($result, CURLOPT_RETURNTRANSFER, true);
// image file
$file_contents = base64_encode(file_get_contents($path));
$request = ‘{
“requests”: [
{
“image”: {
“content”: “‘. $file_contents .'”
},
“features”: [
{
“type”: “LABEL_DETECTION”
}
]
}
]
}’;
curl_setopt($result, CURLOPT_POSTFIELDS, $request);
// curl url
curl_setopt($result, CURLOPT_URL, “https://vision.googleapis.com/v1/images:annotate?key=**********************************************************”);
// post
curl_setopt($result, CURLOPT_CUSTOMREQUEST, ‘POST’);
// –data-binary
curl_setopt($result, CURLOPT_BINARYTRANSFER, true);
// header
curl_setopt($result, CURLOPT_HTTPHEADER, array( “Content-Type: application/json” ));
// get response with text
curl_setopt($result, CURLOPT_RETURNTRANSFER, true);
// image file
$file_contents = base64_encode(file_get_contents($path));
$request = ‘{
“requests”: [
{
“image”: {
“content”: “‘. $file_contents .'”
},
“features”: [
{
“type”: “LABEL_DETECTION”
}
]
}
]
}’;
curl_setopt($result, CURLOPT_POSTFIELDS, $request);
// result
$response = curl_exec($result);
$response = curl_exec($result);
//結果表示
echo “</br>”;
echo “———————————-“;
echo “</br>”;
echo $response ;
echo “</br>”;
echo “———————————-“;
echo “</br>”;
echo “</br>”;
echo “———————————-“;
echo “</br>”;
echo $response ;
echo “</br>”;
echo “———————————-“;
echo “</br>”;
?></p>
</body>
</html>
=======================
</body>
</html>
=======================
結果は、(ペンギンの画像だけど。。。)
いい感じかな???bird、、、とかって言ってるので。。。
いい感じかな???bird、、、とかって言ってるので。。。
(図2)
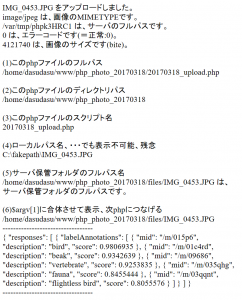
これが、転送した図
(図3)
(図3)
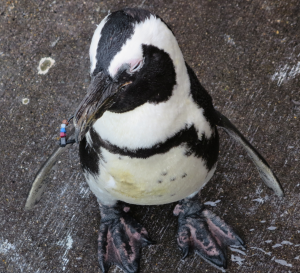
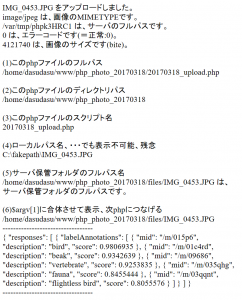
まあ、良い経験できた、、と。
使いすぎないように注意!!!
使いすぎないように注意!!!
以上